MERN Stack Developer Interview Guide
Introduction for MERN Stack Developer Interview Guide
Welcome to the MERN Stack Developer Interview Guide ! Whether you’re a seasoned developer looking to brush up on your skills or preparing for your first MERN stack developer interview guide , this comprehensive guide will provide you with the knowledge and insights you need to succeed.
The MERN stack, composed of MongoDB, Express.js, React.js, and Node.js, has become increasingly popular for building modern web applications. Its versatility, efficiency, and full-stack JavaScript capabilities make it a powerful choice for developers worldwide.
In this guide, we’ll cover a wide range of topics, starting from the foundational concepts of the MERN stack to more advanced techniques and best practices. You’ll learn about front-end development with React.js, back-end development with Node.js and Express.js, database management with MongoDB, as well as additional tools and concepts relevant to MERN stack Developer Interview Guide.
Each section of this guide is carefully structured to provide you with a clear understanding of key concepts and practical insights into real-world scenarios. You’ll find interview questions accompanied by detailed answers, along with explanations and examples to help reinforce your understanding.
Whether you’re preparing for a technical interview, seeking to enhance your MERN stack skills, or simply eager to explore the world of full-stack development, this guide is your comprehensive resource for success.
Let’s dive in and embark on this exciting journey through the MERN Stack Developer Interview Guide !
- MERN Stack Developer Interview Guide
- 1. General Concepts
- 1.1 What is the MERN stack, and how does it differ from other stacks?
- 1.2 Can you explain the role of MongoDB, Express.js, React.js, and Node.js in the MERN stack?
- 2. Frontend (React.js)
- 2.1 What are components in React, and how do you create them?
- 2.2 What is the virtual DOM, and why is it used in React?
- 2.3 How do you handle state in React, and what are the different ways to update it?
- 2.4 Explain the lifecycle methods in React and when you would use them.
- 2.5 What are React hooks, and how do they differ from class components?
- 3. Backend (Node.js & Express.js)
- 4. Database (MongoDB)
- 5. Additional Tools & Concepts
- 5.1 What is JWT, and how do you use it for authentication?
- 5.2 Explain CORS and how to handle it in a MERN application.
- 5.3 What is Docker, and how can it be used in a MERN project?
- 5.4 How do you deploy a MERN application to production?
- 5.5 What are some common security vulnerabilities in MERN applications, and how can you mitigate them?
- 6. Project Experience & Problem-Solving
- 6.1 Can you describe a MERN project you’ve worked on? What challenges did you face, and how did you overcome them?
- 6.2 How do you optimize the performance of a MERN application?
- 6.3 Have you integrated any third-party APIs with your MERN applications? If so, how?
- 6.4 How do you handle errors and debugging in a MERN application?
1. General Concepts
1.1 What is the MERN stack, and how does it differ from other stacks?
- Answer: The MERN stack is a collection of JavaScript-based technologies used to develop web applications. It comprises MongoDB (a NoSQL database), Express.js (a web application framework), React.js (a JavaScript library for building user interfaces), and Node.js (a JavaScript runtime environment). Unlike traditional server-side technologies like LAMP (Linux, Apache, MySQL, PHP), the MERN stack allows for the development of full-stack JavaScript applications, enabling seamless data flow between the client and server.
1.2 Can you explain the role of MongoDB, Express.js, React.js, and Node.js in the MERN stack?
- Answer:
- MongoDB: MongoDB is a NoSQL database that stores data in a flexible, JSON-like format. It serves as the persistent data storage layer in MERN applications, allowing developers to store and retrieve data efficiently.
- Express.js: Express.js is a web application framework for Node.js. It simplifies the process of building web applications and APIs by providing a robust set of features for routing, middleware, and HTTP utilities.
- React.js: React.js is a JavaScript library for building user interfaces. It enables developers to create reusable UI components and manage application state efficiently using a virtual DOM.
- Node.js: Node.js is a JavaScript runtime environment that allows developers to run JavaScript code on the server-side. It provides an event-driven, non-blocking I/O model that makes it ideal for building scalable, real-time applications.
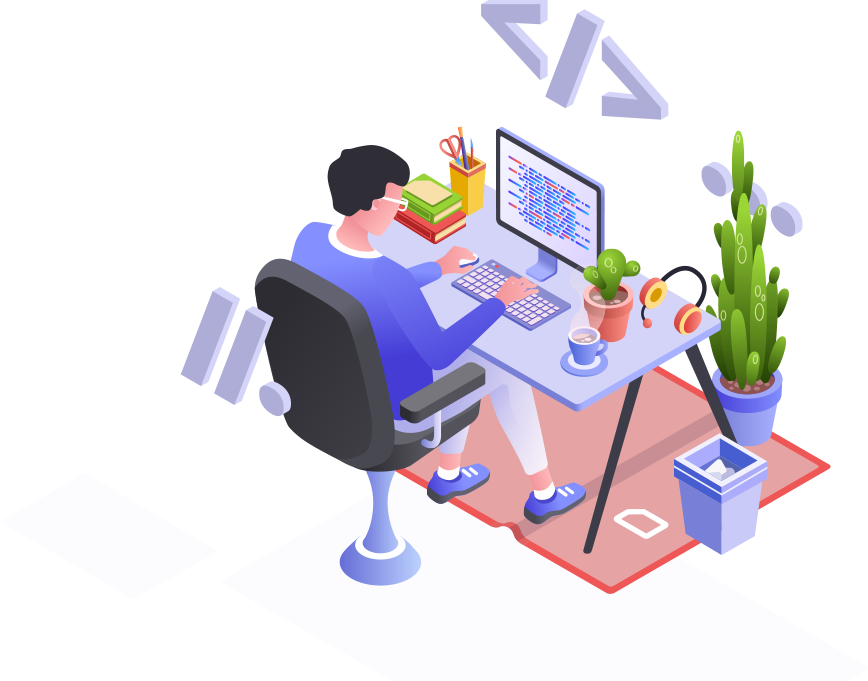
2. Frontend (React.js)
2.1 What are components in React, and how do you create them?
- Answer:
- Components in React are reusable UI elements that encapsulate a piece of functionality and can be composed together to build complex user interfaces.
- Components can be created using either function components or class components. Function components are simpler and use a functional approach, while class components have additional features such as state and lifecycle methods.
2.2 What is the virtual DOM, and why is it used in React?
- Answer:
- The virtual DOM is a lightweight, in-memory representation of the actual DOM (Document Object Model) tree.
- React uses the virtual DOM to perform efficient updates to the UI. When state or props change in a React component, React reconciles the virtual DOM with the actual DOM and updates only the parts of the UI that have changed, resulting in better performance and a smoother user experience.
2.3 How do you handle state in React, and what are the different ways to update it?
- Answer:
- State in React is an object that represents the data specific to a component. It can be accessed and modified using the use State hook (for functional components) or by extending the Component class and using this. state (for class components).
- State can be updated using the set State function (for class components) or by calling the updater function returned by use State (for functional components).
2.4 Explain the lifecycle methods in React and when you would use them.
- Answer:
- React class components have several lifecycle methods that are called at different points in the component’s lifecycle, such as component Did Mount, component Did Update, and component Will Unmount.
- These lifecycle methods allow developers to perform tasks such as fetching data from a server, updating the UI in response to state changes, and cleaning up resources when a component is unmounted.
2.5 What are React hooks, and how do they differ from class components?
- Answer:
- React hooks are functions that allow functional components to use state and other React features without writing a class.
- Hooks were introduced in React 16.8 to address the complexity of managing state and lifecycle methods in class components. They provide a more concise and intuitive way to work with state and side effects in functional components.
3. Backend (Node.js & Express.js)
3.1 What is Node.js, and why is it used in the MERN stack?
- Answer:
- Node.js is a JavaScript runtime environment that allows developers to run JavaScript code on the server-side.
- In the MERN stack, Node.js is used as the backend runtime environment for building server-side logic and handling HTTP requests.
3.2 Explain the concept of middleware in Express.js.
- Answer:
- Middle ware functions in Express.js are functions that have access to the request and response objects and the next middle ware function in the application’s request-response cycle.
- Middle ware functions can perform tasks such as logging, authentication, and error handling before passing control to the next middle ware function in the chain.
3.3 How do you handle routing in Express.js?
- Answer:
- Express.js provides a built-in router that allows developers to define routes for handling HTTP requests.
- Routes in Express.js are defined using the app.get, app.post, app.put, app.delete, etc., methods, which specify the HTTP method and the URL pattern for the route.
3.4 What is a Restful API, and how do you implement it in Express.js?
- Answer:
- Restful API (Representational State Transfer) is an architectural style for designing networked applications, where resources are represented as URLs (Uniform Resource Identifiers), and actions are performed using standard HTTP methods (GET, POST, PUT, DELETE).
- In Express.js, you can implement a Restful API by defining routes that correspond to different resources and using the appropriate HTTP methods to perform CRUD (Create, Read, Update, Delete) operations on those resources.
3.5 How do you connect MongoDB with Node.js?
- Answer:
- MongoDB can be connected to Node.js using the official MongoDB Node.js driver or popular ODM (Object-Document Mapping) libraries such as Mongoose.
- With the MongoDB Node.js driver, you can use the Mongo Client class to establish a connection to the MongoDB server and perform database operations using the provided methods.
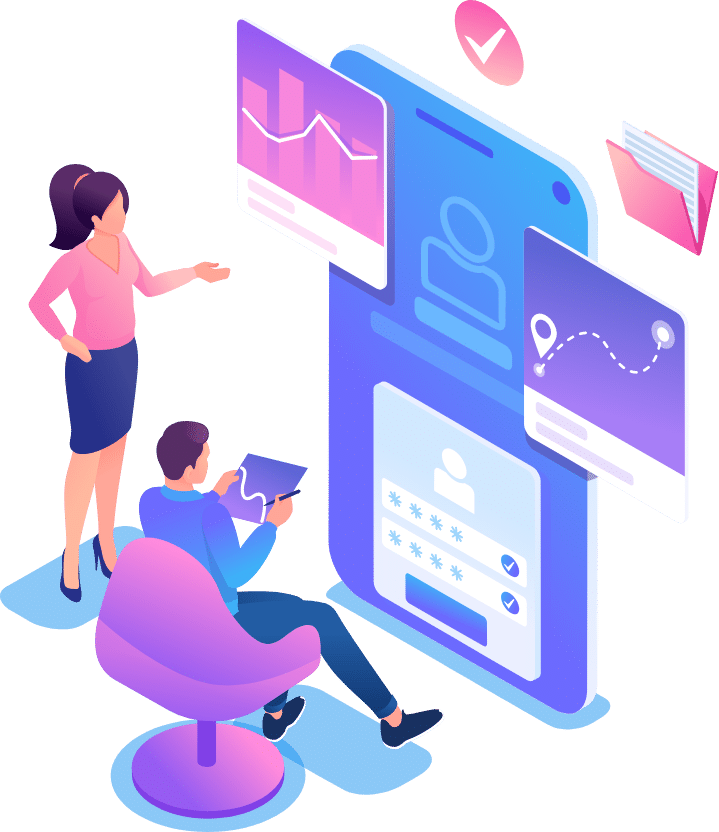
4. Database (MongoDB)
4.1 What is MongoDB, and why would you use it in the MERN stack?
- Answer:
- MongoDB is a NoSQL database that stores data in a flexible, JSON-like format called BSON (Binary JSON).
- In the MERN stack, MongoDB is used as the database layer for storing and retrieving data in a scalable and efficient manner.
4.2 Explain the differences between SQL and NoSQL databases.
- Answer:
- SQL databases (such as MySQL, PostgreSQL) are relational databases that store data in tables with predefined schemas and support ACID (Atomicity, Consistency, Isolation, Durability) transactions.
- NoSQL databases (such as MongoDB, Couch DB) are non-relational databases that store data in flexible, schema-less formats and are optimized for horizontal scalability and high availability.
4.3 How do you perform CRUD operations in MongoDB?
- Answer:
- CRUD (Create, Read, Update, Delete) operations in MongoDB are performed using the insert One, find One, update One, and delete One methods, respectively.
- These methods allow developers to create, read, update, and delete documents in MongoDB collections.
4.4 What is indexing in MongoDB, and why is it important?
- Answer:
- Indexing in MongoDB is the process of creating indexes on fields in a collection to improve query performance.
- Indexes allow MongoDB to quickly locate documents in a collection based on the values of indexed fields, reducing the time required to execute queries.
4.5 How do you handle data consistency and integrity in MongoDB?
- Answer:
- MongoDB provides support for data consistency and integrity through features such as document validation, transactions, and atomic operations.
- Document validation allows developers to enforce schema validation rules on documents in a collection, ensuring data integrity.
- Transactions in MongoDB allow developers to perform multiple operations on multiple documents in a collection in an atomic manner, ensuring data consistency.
5. Additional Tools & Concepts
5.1 What is JWT, and how do you use it for authentication?
- Answer:
- JWT (JSON Web Token) is a compact, URL-safe token format for securely transmitting information between parties as a JSON object.
- In MERN applications, JWT is commonly used for authentication by generating a token containing user information (such as user ID and role) upon successful login and verifying the token on subsequent requests to protected routes.
5.2 Explain CORS and how to handle it in a MERN application.
- Answer:
- CORS (Cross-Origin Resource Sharing) is a security feature implemented by web browsers to restrict requests from one origin to another.
- In a MERN application, CORS can be enabled on the server-side (using middleware like cors in Express.js) to allow or restrict cross-origin requests based on predefined policies.
5.3 What is Docker, and how can it be used in a MERN project?
- Answer:
- Docker is a platform for building, shipping, and running applications in containers.
- In a MERN project, Docker can be used to containerize the application components (such as the front-end, back-end, and database) and deploy them as lightweight, portable containers across different environments.
5.4 How do you deploy a MERN application to production?
- Answer:
- Deploying a MERN application to production typically involves several steps, including building the front end and back end bundles, configuring the server environment, setting up a production database, and deploying the application to a hosting provider (such as AWS, Heroku, or Digital Ocean).
5.5 What are some common security vulnerabilities in MERN applications, and how can you mitigate them?
- Answer:
- Common security vulnerabilities in MERN applications include XSS (Cross-Site Scripting), CSRF (Cross-Site Request Forgery), SQL Injection, and insecure authentication and authorization mechanisms.
- To mitigate these vulnerabilities, developers can implement security best practices such as input validation, output encoding, secure authentication mechanisms (such as JWT), and HTTPS encryption.

6. Project Experience & Problem-Solving
6.1 Can you describe a MERN project you’ve worked on? What challenges did you face, and how did you overcome them?
- Answer:
- Describe a MERN project you’ve worked on, highlighting the technologies used, the role you played, and any challenges you encountered during the development process.
- Discuss how you overcome those challenges by leveraging your problem-solving skills, collaborating with team members, and seeking help from online resources or communities.
6.2 How do you optimize the performance of a MERN application?
- Answer:
- Discuss strategies for optimizing the performance of a MERN application, such as code splitting, lazy loading, server-side rendering, caching, and minimizing network requests.
- Highlight specific techniques you’ve used to improve the performance of your projects and the impact they had on the overall user experience.
6.3 Have you integrated any third-party APIs with your MERN applications? If so, how?
- Answer:
- Describe any third-party APIs you’ve integrated with your MERN applications, explaining the purpose of the integration and the steps involved in implementing it.
- Discuss any challenges you encountered during the integration process and how you resolved them to ensure seamless communication between your application and the external API.
6.4 How do you handle errors and debugging in a MERN application?
- Answer:
- Explain your approach to error handling and debugging in a MERN application, including techniques for identifying and fixing common errors such as syntax errors, runtime errors, and logical errors.
- Discuss the tools and utilities you use for logging, monitoring, and debugging, such as console.log, debugger statements, browser developer tools, and third-party debugging tools.